Java J2ME JSP J2EE Servlet Android
ORA-14552: cannot perform a DDL, commit or rollback inside a query or DML
=====
DML operations like create, drop etc. and rollback/commit can not be performed in a SQL statement
Example:
=======
The most general case where this error occurs is calling a function as
SELECT myFunc(.. .. ..) FROM dual..
Something like this or the like, Where myFunc contains DML operations, rollback or commit statements.
Solution:
==========
You have to ensure that DML operations and rollback/commit operations must be autonomous instead of inside a query.
For the case described above you can execute the function myFunc as
var:=myFunc(.. .. ..);
Or redefine the function as
CREATE OR REPLACE function myFunc(.. .. ..) RETURN ..
AS
....
....
PRAGMA AUTONOMOUS_TRANSACTION
begin
.....
.....
.....
end;
Java By Example: Java Calendar day, month, week, year, hour getting, java.util.Calendar
Java By Example: Java Menue Example [ javax.swing.JMenu ]
Here is a very basic example of Java Menu[JMenue]
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
public class Menu extends JFrame{
public Menu()
{
super("Menu example");
JMenu file = new JMenu("File");
file.setMnemonic(’F’);
JMenuItem newItem = new JMenuItem("New");
newItem.setMnemonic(’N’);
file.add(newItem);
JMenuItem openItem = new JMenuItem("Open");
openItem.setMnemonic(’O’);
file.add(openItem);
JMenuItem exitItem = new JMenuItem("Exit");
exitItem.setMnemonic(’x’);
file.add(exitItem);
//adding action listener to menu items
newItem.addActionListener(
new ActionListener() {
public void actionPerformed(ActionEvent e)
{
System.out.println("New is pressed");
}
}
);
openItem.addActionListener(
new ActionListener(){
public void actionPerformed(ActionEvent e)
{
System.out.println("Open is pressed");
}
}
);
exitItem.addActionListener(
new ActionListener(){
public void actionPerformed(ActionEvent e)
{
System.out.println("Exit is pressed");
}
}
);
JMenuBar bar = new JMenuBar();
setJMenuBar(bar);
bar.add(file);
getContentPane();
setSize(400, 400);
setVisible(true);
}
public static void main(String[] args)
{
Menu app = new Menu();
app.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
Java Vector to array or List to array
Vector friends = new Vector();
friends.add("Tuhin");
friends.add("Sumon");
friends.add("Habib");
friends.add("Shishir");
friends.add("Kiser");
friends.add("Zimi");
friends.add("Lizzie");
String arr[] = (String[]) friends.toArray(new String[0]);
System.out.println(Arrays.toString(arr));
List friendsList = new ArrayList();
friendsList.add("Tuhin");
friendsList.add("Sumon");
friendsList.add("Habib");
friendsList.add("Shishir");
friendsList.add("Kiser");
friendsList.add("Zimi");
friendsList.add("Lizzie");
arr = (String[]) friendsList.toArray(new String[0]);
System.out.println(Arrays.toString(arr));
If you execute the above code block. This will generate an output as follows..
[Tuhin, Sumon, Habib, Shishir, Kiser, Zimi, Lizzie]
[Tuhin, Sumon, Habib, Shishir, Kiser, Zimi, Lizzie]
Java Sorting Array, List
String friends[] = {"Tuhin", "Sumon","Habib","Shishir","Kiser","Zimi","Lizzie"};
System.out.println(Arrays.toString(friends));
List tempList = Arrays.asList(friends);
System.out.println(tempList);
Collections.sort(tempList);
System.out.println(tempList);
friends = (String[]) tempList.toArray(new String[0]);
System.out.println(Arrays.toString(friends));
This code block if execute will generate output like ...
[Tuhin, Sumon, Habib, Shishir, Kiser, Zimi, Lizzie]
[Tuhin, Sumon, Habib, Shishir, Kiser, Zimi, Lizzie]
[Habib, Kiser, Lizzie, Shishir, Sumon, Tuhin, Zimi]
[Habib, Kiser, Lizzie, Shishir, Sumon, Tuhin, Zimi]
ORACLE: Getting maximum sub string
CREATE table test(
name varchar2(100),
code varchar2(50)
)
INSERT INTO test(name,code) VALUES('Bangladesh','880');
INSERT INTO test(name,code) VALUES('BGD-Mobile','8801');
INSERT INTO test(name,code) VALUES('BGD-GP','88017');
INSERT INTO test(name,code) VALUES('BGD-AKTEL','88018');
INSERT INTO test(name,code) VALUES('BGD-Bangla Link','88019');
INSERT INTO test(name,code) VALUES('BGD-CITYCELL','88011');
INSERT INTO test(name,code) VALUES('BGD-WARID','88016');
INSERT INTO test(name,code) VALUES('BGD-TELETALK','88015');
SELECT * FROM test
SELECT max(code) FROM test WHERE instr('8801234',code)>0;
This will give: 8801
SELECT max(code) FROM test WHERE instr('880234',code)>0;
This will give: 880
SELECT max(code) FROM test WHERE instr('8801123',code)>0;
This will give: 88011
ORA-01704: String literal too long
In Java if you try to execute PreparedStatement.executeUpdate() to INSERT or UPDATE CLOB field of ORACLE with more than 4000 characters, you'll get this error.
To avoid this use PreparedStatement.execute() methode to to INSERT CLOB field.
This may occur in other language also. And you can use same technique to avoid errors.
Happy programming.
Comment my blog/ email me for any problems related to this.
Java by Example: Exception Handling, creating custom exception class
Java by Example: Exception Handling, creating custom exception class
Java by Example: Exception handling – throw custom Exception
Java By Example: Exception Handling – Throwing exception from method
Java by Example: Exception handling – Basic Exception Handling example ‘try catch’
Java by Example: Exception Handling – Java Exception Basics
You can easily create your own Exception Class where you can put your own exception. To do this what you have to do is just you have create an subclass of java.lang.Exception class.
Here is a basic example of creating custom exception class
package example.java.lang;
public
class
ExceptionExp5
extends Exception{
public ExceptionExp5() {
super("[ MY EXCEPTION ] ");
}
public ExceptionExp5(String exceptionString) {
super("[ MY EXCEPTION ] "+exceptionString);
}
public
static
void main(String args[]){
try{
new
ExceptionExp5().tryCustomException();
}catch(Exception e){
e.printStackTrace();
}
}
public
void tryCustomException() throws Exception{
int fibs[]={1,2,3,5,8,10,13};
for(int i=0;i<fibs.length;i++){
if(isFibs(fibs[i]))
System.out.println(fibs[i]+" - OK");
else
throw
new
ExceptionExp5("Invalid fibonacci number "+fibs[i]);
}
}
private
boolean isFibs(int num){
int f0 = 1;
int f1 = 1;
int fib = 1;
while(num>=fib){
if(num==fib)
return
true;
fib = f0 + f1;
f0 = f1;
f1 = fib;
}
return
false;
}
}
If you execute this you'll get output like..
1 - OK
2 - OK
3 - OK
5 - OK
8 - OK
example.java.lang.ExceptionExp5: [ MY EXCEPTION ] Invalid fibonacci number 10
at example.java.lang.ExceptionExp5.tryCustomException(ExceptionExp5.java:23)
at example.java.lang.ExceptionExp5.main(ExceptionExp5.java:12)
Java by Example: Exception handling – throw custom Exception
Java by Example: Exception Handling, creating custom exception class
Java by Example: Exception handling – throw custom Exception
Java By Example: Exception Handling – Throwing exception from method
Java by Example: Exception handling – Basic Exception Handling example ‘try catch’
Java by Example: Exception Handling – Java Exception Basics
Exception indicates illegal operation. So for your program you can define an operation as illegal. Say in your login program you allow maxim 3 times retry with wrong user/password. Then you can define your exception for attempt to login for more than thrice. And if this case occurs you can throw your exception.
Here is an example of throw custom exception to check if a number is a Fibonacci number or not. When a non Fibonacci number found exception 'Invalid fibonacci number' will be thrown..
Here is the example . .
public
class ExceptionExp4 {
public
static
void main(String args[]){
ExceptionExp4 excpExp = new ExceptionExp4();
try{
excpExp.tryCustomException();
}catch(Exception ce){
ce.printStackTrace();
}
}
public
void tryCustomException() throws Exception{
int fibs[]={1,2,3,5,8,10,13};
for(int i=0;i<fibs.length;i++){
if(isFibs(fibs[i]))
System.out.println(fibs[i]+" - OK");
else
throw
new Exception("Invalid fibonacci number "+fibs[i]);
}
}
private
boolean isFibs(int num){
int f0 = 1;
int f1 = 1;
int fib = 1;
while(num>=fib){
if(num==fib)
return
true;
fib = f0 + f1;
f0 = f1;
f1 = fib;
}
return
false;
}
}
Fibonacci number series is
1 2 3 5 8 13 21 34 . . . .
So if you try to execute exception should occur when 10 is found..
Lets see the output of this program...
1 - OK
2 - OK
3 - OK
5 - OK
8 - OK
java.lang.Exception: Invalid fibonacci number 10
at example.java.lang.ExceptionExp4.tryCustomException(ExceptionExp4.java:18)
at example.java.lang.ExceptionExp4.main(ExceptionExp4.java:7)
So in this way we can easily create our own exception with our own message as per our needs.
Java By Example: Exception Handling – Throwing exception from method
Java by Example: Exception Handling, creating custom exception class
Java by Example: Exception handling – throw custom Exception
Java By Example: Exception Handling – Throwing exception from method
Java by Example: Exception handling – Basic Exception Handling example ‘try catch’
Java by Example: Exception Handling – Java Exception Basics
The second way of exception handling is throwing the exception. This is done by adding throws with method name.
Syntax of throwing exception from method is
access_modifier return_type method_name(arguments) throws exception_list{
}
Here is a practical example of doing this..
public
class ExceptionExp3 {
public
static
void main(String args[]){
ExceptionExp3 excpExp = new ExceptionExp3();
try{
excpExp.tryThrows();
}catch(ArrayIndexOutOfBoundsException ae){
System.out.println("Exception Caught");
}
}
public
void tryThrows() throws ArrayIndexOutOfBoundsException{
String strArray[] = {"This","is", "an", "example", "of", "Java","exception" };
System.out.println(strArray[0]);
System.out.println(strArray[1]);
System.out.println(strArray[3]);
System.out.println(strArray[4]);
System.out.println(strArray[5]);
System.out.println(strArray[6]);
System.out.println(strArray[7]);
System.out.println("This statement wont execute");
}
}
The ArrayIndexOutOfBoundsException is thrown from method tryThrows() instead of handling the exception. In this case when exception occurred in tryThrows() method instead of terminating the program this method throw the exception to caller.
This program will give the following output..
This
is
example
of
Java
exception
Exception Caught
Java by Example: Exception handling – Basic Exception Handling example ‘try catch’
Java by Example: Exception Handling, creating custom exception class
Java by Example: Exception handling – throw custom Exception
Java By Example: Exception Handling – Throwing exception from method
Java by Example: Exception handling – Basic Exception Handling example ‘try catch’
Java by Example: Exception Handling – Java Exception Basics
Exception Handling: The basic way to cope with exception is using try ...catch.
The Syntax for using try...catch is
try{
...
...
Code have to monitor for exception
...
...
}catch(Exception e){
...
Exception action code
...
}
Here is an easy example to demonstrate the above case..
public
class ExceptionExp2 {
public
static
void main(String args[]){
String strArray[] = {"This","is","an","example","of","Java","exception" };
try{
System.out.println(strArray[0]);
System.out.println(strArray[1]);
System.out.println(strArray[3]);
System.out.println(strArray[4]);
System.out.println(strArray[5]);
System.out.println(strArray[6]);
System.out.println(strArray[7]);
}catch(ArrayIndexOutOfBoundsException exp){
System.out.println("Exception Occurred");
}
System.out.println("This will execute");
}
}
If you run the above code it will give the following output …
This
is
example
of
Java
exception
Exception Occurred
This will execute
So exception is handled here by printing a message "Exception Occurred" and the program continues to execute the codes after the try ... catch block instead of terminating.
Java by Example: Exception Handling – Java Exception Basics
Java by Example: Exception Handling, creating custom exception class
Java by Example: Exception handling – throw custom Exception
Java By Example: Exception Handling – Throwing exception from method
Java by Example: Exception handling – Basic Exception Handling example ‘try catch’
Java by Example: Exception Handling – Java Exception Basics
Java provides strong way to cope with Errors and Exceptions. When an illegal operation occurred Java throws exception. And When exception occurs program execution ends if exception is not handled properly. There is a class below to understand Java Exception..
public
class ExceptionExp1 {
public
static
void main(String args[]){
String strArray[] = {"This","is", "an", "example", "of", "Java","exception" };
System.out.println(strArray[0]);
System.out.println(strArray[1]);
System.out.println(strArray[3]);
System.out.println(strArray[4]);
System.out.println(strArray[5]);
System.out.println(strArray[6]);
System.out.println(strArray[7]);
System.out.println("Program will terminate before executing this line");
}
}
If you execute this program, the output will be-
This
is
example
of
Java
exception
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 7
at example.java.lang.ExceptionExp1.main(ExceptionExp1.java:13)
Here exception occurs before ends the program and terminates.
Oracle : Hierarchical Data/ Tree in Oracle
The syntax for query hierarchical data is-
SELECT columns
FROM table_name
WHERE where_condition
CONNECT BY PRIOR condition_for_next_level
START WITH start_condition
ORDER SIBLINGS BY columns
Let's try it with a practical example.
CREATE
TABLE location(
id NUMBER,
name
VARCHAR2(100),
parent NUMBER
);
INSERT
INTO location(id,name,parent) VALUES(1,'Earth',0);
INSERT
INTO location(id,name,parent) VALUES(2,'Asia',1);
INSERT
INTO location(id,name,parent) VALUES(3,'Europe',1);
INSERT
INTO location(id,name,parent) VALUES(4,'America',1);
INSERT
INTO location(id,name,parent) VALUES(5,'Africa',1);
INSERT
INTO location(id,name,parent) VALUES(6,'Oceania',1);
INSERT
INTO location(id,name,parent) VALUES(7,'Bangladesh',2);
INSERT
INTO location(id,name,parent) VALUES(8,'India',2);
INSERT
INTO location(id,name,parent) VALUES(9,'Pakistan',2);
INSERT
INTO location(id,name,parent) VALUES(10,'Singapore',2);
INSERT
INTO location(id,name,parent) VALUES(11,'Malaysia',2);
INSERT
INTO location(id,name,parent) VALUES(12,'England',3);
INSERT
INTO location(id,name,parent) VALUES(13,'France',3);
INSERT
INTO location(id,name,parent) VALUES(14,'Turkey',3);
INSERT
INTO location(id,name,parent) VALUES(15,'Scotland',3);
INSERT
INTO location(id,name,parent) VALUES(16,'Mexico',4);
INSERT
INTO location(id,name,parent) VALUES(17,'Canada',4);
INSERT
INTO location(id,name,parent) VALUES(18,'USA',4);
INSERT
INTO location(id,name,parent) VALUES(19,'Dhaka',7);
INSERT
INTO location(id,name,parent) VALUES(20,'Rajshahi',7);
INSERT
INTO location(id,name,parent) VALUES(21,'Chittagong',7);
INSERT
INTO location(id,name,parent) VALUES(22,'Las Vegas',18);
INSERT
INTO location(id,name,parent) VALUES(23,'New Orlince',18);
INSERT
INTO location(id,name,parent) VALUES(24,'New York',18);
Now
SELECT * FROM location;
Will give..
ID NAME PARENT
-----------------------------------------------
1 Earth 0
2 Asia 1
3 Europe 1
4 America 1
5 Africa 1
6 Oceania 1
7 Bangladesh 2
8 India 2
9 Pakistan 2
10 Singapore 2
11 Malaysia 2
12 England 3
13 France 3
14 Turkey 3
15 Scotland 3
16 Mexico 4
17 Canada 4
18 USA 4
19 Dhaka 7
20 Rajshahi 7
21 Chittagong 7
22 Las Vegas 18
23 New Orlince 18
24 New York 18
Now
SELECT
lpad(' ',8*(level-1))||NAME AS name
FROM LOCATION
CONNECT
BY
PRIOR id = parent
START
WITH parent = 0
Will give the following result
NAME
------------
Earth
Asia
Bangladesh
Dhaka
Rajshahi
Chittagong
India
Pakistan
Singapore
Malaysia
Europe
England
France
Turkey
Scotland
America
Mexico
Canada
USA
Las Vegas
New Orlince
New York
Africa
Oceania
You can also use ORDER SIBLINGS BY to sort siblings
ORACLE - ALTER TABLE: Rename Table
ALTER TABLE table_name
RENAME TO new_table_name;
Example:
ALTER TABLE emp
RENAME TO emp_records;
This will change the table name from 'emp' to emp_record.
Oracle : ALTER TABLE: Add Columns
Syntax:
ALTER
TABLE
table_name
ADD(
column1 datatype1 constraint1,
column2 datatype2 constraint2,
column3 datatype3 constraint3
);
ALTER
TABLE
emp
ADD(
designation VARCHAR2(100) UNIQUE,
rank NUMBER
NOT
NULL
);
This will add 2 columns to the table 'emp' named 'designation' and 'rank' with given constraints and data types
Java Difference between Hashtable and Hashmap / Hashtable versus Hashmap
The majore differences are described below...
1. Synchronous/asynchronous: Hashtable is synchronous and Hashmap is not synchronous by default.
2. null value as key: Hashtable does not support null value as hash key but Hasmap support it.
3. Faile-safe: Iterator of Hashmap is fail-safe but Enumeration of Hashtable is not failsafe.
Perhaps this will be able to eliminate any misconception about Hashtable and Hashmap
Java Dangling meta character, PatternSyntaxException
PatternSyntaxException: Unmatched closing ')',
PatternSyntaxException: Unclosed group near,
PatternSyntaxException: Dangling meta character
The cause of the above Exceptions are you're trying to use meta character in Java Pattern processing, like regular expression.
The meta characters in this case are ([{\^-$|]})?*+
So if you want to process a String containing any of the meta characters in regular expression procession using java regex you'll get Exception like above. To process String containing a meta character just use escape character, a fore slash('/') before that character.
Any java method that use java regex also give such errors.
For example
Let's see the code below
String input = "I live in Dhaka(agargaon), Bangladesh";
String arr[] = input.split(")");
This will give the Exception PatternSyntaxException: Unmatched closing ')',
One more such method is indexOf().
In this case you have ti change the above code as
String arr[] = input.split("\\)");
Or for indexOf
input.indexOf("\\)");
Using cookie in Java servlet/ jsp
You can easily set cookie by the following way..
<%
String myName=request.getParameter("myName");
if(myName==null) myName="";
Date now = new Date();
String timestamp = now.toString();
Cookie cookie = new Cookie ("myName",myName);
cookie.setMaxAge(2 * 365 * 24 * 60 * 60);
response.addCookie(cookie);
%>
Here a cookie name 'myName' is created with value request.getParameter("myName"). The lifetime for this cookie is set to 2 years;
You you can fetch that cookie using jsp/servlet easily by the following way
<%
String cookieName = "myName";
Cookie allCookies [] = request.getCookies ();
Cookie cook = null;
if (allCookies != null)
{
for (int i = 0; i < allCookies.length; i++)
{
if (allCookies [i].getName().equals (cookieName))
{
cook = allCookies[i];
break;
}
}
}
%>
The Cookie object cook will contain the requested cookie. And you can get the value of the cookie by calling cook.getValue() methode.
Thank you.
Using Session in JSP
See the following code...
<%
Vector users = new Vector();
users.add("ABC");
users.add("BCD");
users.add("CDE");
session.setAttribute("users", users);
%>
This will set the Vector object users to session with name users.
To get values from session we can do the following..
<%
Vector users = (Vector)getAttribute("users");
for(int i=0;i<users.size();i++)
out.print((String)users.get(i)+"<br >");
%>
In this way we can handle session in JSP/servlet.
For any query just add comment..
Using SVN
To use svn you need an svn client. You can download a good svn client from http://tortoisesvn.tigris.org/ you can also add an cvs client for eclipse named subclipse from the following eclipse update site
Name: Subclipse 1.4.x (Eclipse 3.2+)
URL: http://subclipse.tigris.org/update_1.4.x
Name: Subclipse 1.2.x (Eclipse 3.2+)
URL: http://subclipse.tigris.org/update_1.2.x
Name: Subclipse 1.0.x (Eclipse 3.0/3.1)
URL: http://subclipse.tigris.org/update_1.0.x
To checkout and import a project from and to SVN use the following parameters..
url: svn://<hostname><repository> eg. svn://localhost/usr/svn/svnroot
If you use authenticated access instead of anonymous access you need user name and password eg. user1 and pass1
This post was published to Silicon Magic at 5:22:42 PM 3/28/2009
[Enter Post Title Here]
- Create repository
Create a svn repository by the command
$ svnadmin create /usr/svn/svnroot
Edit for authentication
Edit [general] section of svnroot/conf/svnserve.conf file as
[general]
anon-access = write
This will allow anonymous user to checkout and commit svn server. To restrict access to user do the following
Edit the [general] section of svnserve.conf as
[general]
anon-access = none
auth-access = write
authz-db = authz
password-db = passwd
- Edit the [/] section of authz file as
[/]
user1 = rw
user2 = r
* =
c. Edit the [user] section of passwd file as
[user]
user1 = pass1
user2 = pass2
Start SVN repository
Start/Initiate repository by the following command
$ svnserve -d -r /usr/svn/svnroot
SVN Installation (on Unix/Linux)
- Prerequisites Installing
- autoconf
- libtool
- a reasonable C compiler (gcc, Visual Studio, etc.
- libapr
- libapr-util
- libz
- libserf
- OpenSSL
Install above prerequisites properly if your system missing any of the above list. If you have tarbal file(XXXX.tar.gz) install as
- autoconf
$ tar xzvf XXXX.tar.gz
$ cd XXXX
$ ./configure
$ make
$ make install
Otherwise if you have rpm(YYYY.rpm) for the above listed prerequisites, install them by the command
$ rpm –i YYYY.rpm
- SVN Installing
Down load SVN tarball file from http://subversion.tigris.org/getting.html#source-release
$ tar xzvf subversion-1.x.x.tar.gz
$ cd subversion-1.x.x
$ ./autogen
$ ./configure --with-apr=/usr/local/apr --with-apr-util=/usr/local/apr
$ make
$ make install
Print a part of a webpage using javascript
We can easily print a part of a webpage by calling window.print() .
Here is an example to print a <div>
<html>
<head>
</head>
<body>
<div style = "width:100%;height:100" id="myDiv">
This is to print<br/>
Testing
</div>
<div style = "width:100%;height:100">
This is not to print<br/>
Testing
</div>
<br/>
<span onClick="printIt();">Click here</span>
<script type = "text/javascript">
function printIt(){
user_pref("print.print_footercenter","");
user_pref("print.print_footerleft","");
user_pref("print.print_footerright","");
user_pref("print.print_headerleft","");
user_pref("print.print_headercenter","");
user_pref("print.print_headerright","");
wi = window.open('', 'p');
wi.document.open();
element=document.getElementById("myDiv");
wi.document.write(element.innerHTML);
wi.print();
wi.document.close();
wi.close();
}
</script>
</body>
</html>
Print a part of a webpage using javascript
We can easily print a part of a webpage by calling window.print() .
Here is an example to print a <div>
<html>
<head>
</head>
<body>
<div style = "width:100%;height:100" id="myDiv">
This is to print<br/>
Testing
</div>
<div style = "width:100%;height:100">
This is not to print<br/>
Testing
</div>
<br/>
<span onClick="printIt();">Click here</span>
<script type = "text/javascript">
function printIt(){
wi = window.open('', 'p');
wi.document.open();
element=document.getElementById("myDiv");
wi.document.write(element.innerHTML);
wi.print();
wi.document.close();
wi.close();
}
</script>
</body>
</html>
Eclipse plug-in for android development
1. Click help from eclipse menue
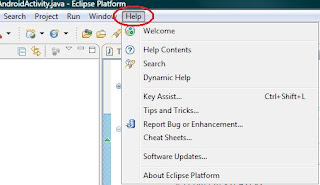
2. Click on Software Updates...
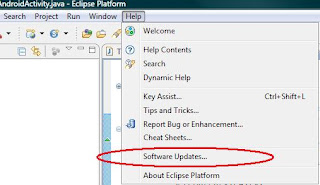
3. A new window will come. Click on Add sites
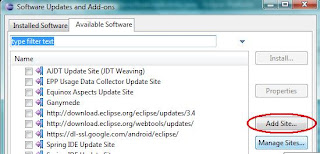
4. Type http://dl-ssl.google.com/android/eclipse/ in location field then press OK.
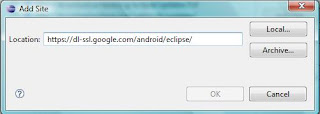
5. Now check the ckeck boxes left to new android update site name. And press Install
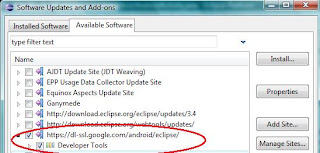
6. Check all check boxex in new popup window and click Finish.
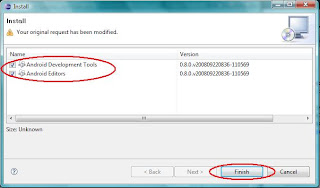
7. Next follow the normal installation steps to complete installation.
Now your eclipse is ready for android software development.